RslArg Class Reference
#include <RslPlugin.h>
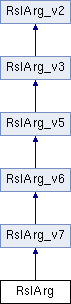
Public Member Functions | |
virtual | ~RslArg () |
Virtual destructor. | |
![]() | |
virtual bool | IsFilterRegion () const =0 |
Returns true if this argument is a filter region. | |
virtual | ~RslArg_v7 () |
Virtual destructor. | |
![]() | |
virtual | ~RslArg_v6 () |
Virtual destructor. | |
![]() | |
virtual bool | IsStruct () const =0 |
Returns true if this argument is a struct (or struct array). | |
virtual const char * | GetName () const =0 |
virtual bool | IsResizable () const =0 |
Returns true if this argument is a resizable array. | |
virtual class RslResizer * | GetResizer () const =0 |
Get resizer for this argument (returns NULL if not resizable). | |
virtual | ~RslArg_v5 () |
Virtual destructor. | |
![]() | |
virtual bool | IsNormal () const =0 |
Returns true if this argument is a normal (or normal array). | |
virtual bool | IsWriteable () const =0 |
virtual | ~RslArg_v3 () |
Virtual destructor. | |
![]() | |
virtual | ~RslArg_v2 () |
Virtual destructor. | |
virtual bool | IsFloat () const =0 |
Returns true if this argument is a float (or float array). | |
virtual bool | IsPoint () const =0 |
Returns true if this argument is a point (or point array). | |
virtual bool | IsVector () const =0 |
Returns true if this argument is a vector (or vector array). | |
virtual bool | IsColor () const =0 |
Returns true if this argument is a color (or color array). | |
virtual bool | IsString () const =0 |
Returns true if this argument is a string (or string array). | |
virtual bool | IsMatrix () const =0 |
Returns true if this argument is a matrix (or matrix array). | |
virtual bool | IsArray () const =0 |
Returns true if this argument is an array. | |
virtual bool | IsVarying () const =0 |
Returns true if this argument is varying. | |
virtual int | GetArrayLength () const =0 |
virtual unsigned int | NumValues () const =0 |
virtual void | GetData (float **data, int *stride) const =0 |
Additional Inherited Members | |
![]() | |
static unsigned int | NumValues (int argc, const class RslArg **argv) |
Detailed Description
An array of RslArg pointers is passed to the RSL plugin function. The result parameter is always the zeroth argument (even in a void function).
Various methods can be used to query the type of an RslArg (e.g. IsFloat(), IsArray()), its detail (IsVarying()), and other information (GetArrayLength(), IsWriteable()).
An iterator (RslIter) must be constructed to access the data represented by an RslArg. The type of iterator depends on the underlying data type. For example:
If an argument might be varying, the NumValues() method is used to determine the number of iterations required to process it. Any arguments that are varying require the same number of iterations. If the function returns a result (i.e. does not have a void return type), the shader compiler guarantees that the result argument (argv[0]) is varying whenever any of the other arguments are varying. It's usually sufficient to use "argv[0]->NumValues()" as the required number of iterations. For example:
Void plugin functions must take the detail of all the arguments into account, however:
Note that incrementing an iterator for a uniform argument has no effect. Also, the number of iterations might be one if all the arguments are uniform. In our experience, it is usually not worth optimizing for such cases. If performance is a concern, overloading can be used to define a specialized plugin function that operates on all uniform arguments.
The documentation for this class was generated from the following file: